views
Playwright Examples: Unlock the Power of Automation and Testing in Web Development
When diving into the world of web development, ensuring that your applications are both robust and reliable is crucial. One of the most powerful tools that have emerged for automating browser interactions is Playwright, a modern testing framework that allows developers to write efficient and effective tests. If you're exploring Playwright for your automation needs, you might be looking for inspiration or understanding how to maximize its potential.
In this article, we will explore some of the best Playwright examples, discussing practical use cases, techniques, and advanced features that can elevate your testing workflow. Whether you're a beginner just starting to explore Playwright or an experienced developer looking for more sophisticated testing methods, these examples will help guide you toward better automation practices. Additionally, we will show how integrating Playwright with tools like Testomat.io can significantly improve your test management process.
What Is Playwright?
Playwright is a Node.js library that enables browser automation. It supports testing across all modern browsers—Chromium, Firefox, and WebKit—allowing for fast, reliable, and scalable end-to-end tests. One of its biggest selling points is its ability to run tests in parallel, which can dramatically speed up the testing process. It’s particularly powerful for performing tests that mimic real user interactions, such as navigating pages, filling out forms, or checking elements.
In this article, we will explore several Playwright examples that showcase its features and use cases. These examples will help you understand how Playwright fits into your web development and testing lifecycle.
1. Introduction to Playwright Testing with Examples
When starting with Playwright, the first step is setting up your testing environment. After installing Playwright, you can quickly write your first test. Below is a simple Playwright example that navigates to a website and verifies the title.
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const title = await page.title();
console.log(title);
await browser.close();
})();
This simple script launches Chromium, navigates to https://example.com
, and prints the page's title. It's a good starting point, but Playwright’s power lies in its ability to interact with complex elements on a webpage, run tests in multiple browsers, and even simulate user behavior like clicks, form submissions, and scrolling.
2. Testing Dynamic Content with Playwright
In modern web development, dynamic content is a common feature. Playwright makes it easy to handle dynamic elements, such as those loaded by JavaScript. In this Playwright example, we will test a dynamic list of items that loads asynchronously.
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com/dynamic-list');
// Wait for the list items to load
await page.waitForSelector('.list-item');
// Get all items from the list
const items = await page.$$eval('.list-item', items => items.map(item => item.textContent));
console.log(items);
await browser.close();
})();
This script waits for the list items to appear on the page before collecting them. Using $$eval
, Playwright can query multiple elements, making it ideal for testing dynamic content that requires waiting for elements to load or change.
3. Advanced Playwright Example: Handling Multiple Browsers
One of the unique features of Playwright is its ability to run tests across multiple browsers simultaneously. This can save a lot of time when testing cross-browser compatibility. In this Playwright example, we demonstrate running tests on both Chromium and WebKit at the same time.
const { chromium, webkit } = require('playwright');
(async () => {
const [chromiumBrowser, webkitBrowser] = await Promise.all([
chromium.launch(),
webkit.launch()
]);
const [chromiumPage, webkitPage] = await Promise.all([
chromiumBrowser.newPage(),
webkitBrowser.newPage()
]);
await Promise.all([
chromiumPage.goto('https://example.com'),
webkitPage.goto('https://example.com')
]);
const chromiumTitle = await chromiumPage.title();
const webkitTitle = await webkitPage.title();
console.log(`Chromium Title: ${chromiumTitle}`);
console.log(`WebKit Title: ${webkitTitle}`);
await Promise.all([chromiumBrowser.close(), webkitBrowser.close()]);
})();
This Playwright example demonstrates how to launch both Chromium and WebKit browsers in parallel and perform tests on them. This cross-browser functionality is especially useful when you need to ensure your web applications work well on different platforms.
4. Playwright Examples for API Testing
Besides browser automation, Playwright also supports testing APIs. You can use Playwright to send HTTP requests and inspect the responses. Here is a Playwright example that demonstrates API testing:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
// Intercept and mock an API request
await page.route('**/api/v1/user', route => {
route.fulfill({
status: 200,
body: JSON.stringify({ username: 'testuser', id: 123 })
});
});
// Navigate to a page that calls the API
await page.goto('https://example.com');
const response = await page.request.get('https://example.com/api/v1/user');
const json = await response.json();
console.log(json);
await browser.close();
})();
This Playwright example demonstrates how you can intercept and mock an API request, allowing you to simulate different API responses during your testing.
5. Test Automation and Management with Testomat.io
When working with Playwright, managing your tests effectively is just as important as writing them. This is where Testomat.io comes into play. Testomat.io is a test management platform that integrates seamlessly with Playwright and other testing frameworks. It allows you to organize, track, and analyze your test results in one centralized location.
Some key benefits of using Testomat.io include:
-
Real-Time Test Reporting: Get instant feedback on your Playwright tests, including detailed logs and visual insights into test performance.
-
Collaboration: Team members can collaborate on tests, view shared test results, and ensure that everyone is aligned on testing efforts.
-
Integration with CI/CD: Automate your testing process with continuous integration tools, streamlining your development lifecycle.
Testomat.io helps you get the most out of your Playwright tests, offering advanced features like analytics, test case management, and seamless reporting.
6. Combining Playwright and Testomat.io: A Powerful Duo
Integrating Playwright examples with Testomat.io for test management enhances your automation experience. By connecting Playwright’s test results directly to Testomat.io, you can gain powerful insights and track the success of your tests across different environments.
To get started, visit Testomat.io for more information on how you can integrate Playwright and Testomat.io to create a seamless testing and management pipeline.
Conclusion
In this article, we’ve covered several Playwright examples that demonstrate the framework’s flexibility and power in automating browser testing. From basic interactions to more advanced use cases like multi-browser testing and API mocking, Playwright offers a comprehensive solution for modern web applications.
By combining Playwright with Testomat.io, you can take your testing efforts to the next level, improving collaboration, test management, and reporting. Playwright examples, combined with the robust features of Testomat.io, can significantly enhance the quality and efficiency of your web development and testing processes.
Visit Testomat.io for more in-depth insights into Playwright repositories and to start managing your tests more effectively today!
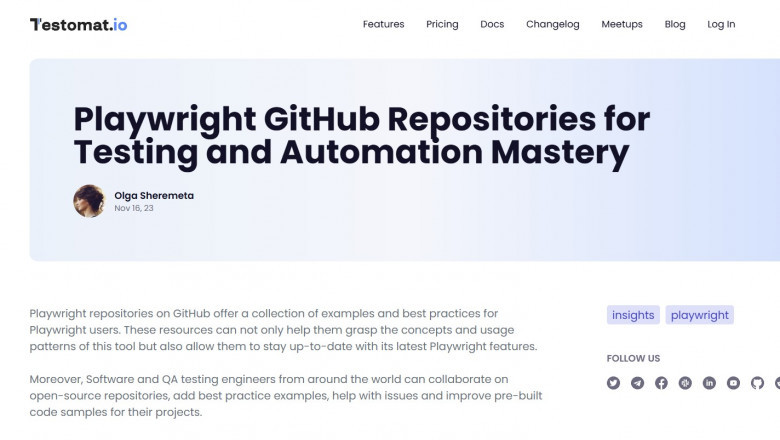

Comments
0 comment